Arithmetic Operators in JavaScript

Arithmetic operators are fundamental to programming in JavaScript, allowing developers to perform basic mathematical operations like addition, subtraction, multiplication, and division. Mastering these operators is essential for handling numerical data in a wide range of applications—from simple calculations to complex algorithms.
In this section, we’ll cover the following topics:
- What Are Arithmetic Operators?
- Use Cases of Arithmetic Operators with Variables
- The + Operator for String and Number
What Are Arithmetic Operators?
Arithmetic operators are fundamental tools in JavaScript that enable numerical
calculations like addition (+
), subtraction (-
),
multiplication (*
), division (/
), modulus
(%
), and exponentiation (**
). These symbols work
with integers and floating-point numbers to manipulate numeric data across
various applications. They are essential for building logic and handling
mathematical operations in JavaScript programs.
The Addition Operator (+)
The addition operator (+
) is used to add two numbers. It can also
be used to concatenate strings, making it versatile in both numerical and
string operations.
Example:
let sum = 5 + 3;
console.log(sum); // Result: 8
In this case, 5
and 3
are added to give a result of
8
.
The Subtraction Operator (-)
The subtraction operator (-
) is used to subtract one number from
another.
Example:
let difference = 10 - 4;
console.log(difference); // Result: 6
Here, 4
is subtracted from 10
, and the result is
6
.
The Multiplication Operator (*)
The multiplication operator (*
) is used to multiply two numbers.
Example:
let product = 6 * 7;
console.log(product); // Result: 42
In this example, 6
is multiplied by 7
to give the
result 42
.
The Division Operator (/)
The division operator (/
) divides one number by another. Be
cautious when dividing by zero, as it will result in Infinity
or
NaN
(Not a Number).
Example:
let quotient = 8 / 2;
console.log(quotient); // Result: 4
Here, 8
is divided by 2
, and the result is
4
.
The Modulus Operator (%)
The modulus operator (%
) returns the remainder of a division
operation. It’s useful for tasks like determining whether a number is even or
odd.
Example:
let remainder = 10 % 3;
console.log(remainder); // Result: 1
In this case, 10
divided by 3
leaves a remainder of
1
.
The Exponentiation Operator (**)
The exponentiation operator (**
) raises a number to the power of
another number.
Example:
let power = 2 ** 3;
console.log(power); // Result: 8
In this example, 2
is raised to the power of 3
,
resulting in 8
.
Use Cases of Arithmetic Operators with Variables
Arithmetic operators are often used with variables to store dynamic results. Let’s look at some practical examples of how you can use these operators in real-world scenarios.
Performing Operations on Variables
In JavaScript, variables are used to store data that can change over time. You can perform arithmetic operations on these variables, allowing you to modify values as needed in your program.
Example:
let a = 10;
let b = 5;
let result = a + b;
console.log(result); // Result: 15
Here, the values of a
and b
are added together and
stored in the result
variable.
Example: Calculating the Area of a Circle
Arithmetic operators can also be used in more complex calculations. For
instance, to calculate the area of a circle, you can use the formula
Area = π * r^2
, where r
is the radius.
Example:
let radius = 5;
let area = Math.PI * radius ** 2;
console.log(area); // Result: 78.5398
In this example, the exponentiation operator is used to square the radius, and
Math.PI
gives the value of π.
Example: Creating a Simple Calculator
A simple calculator program can utilize arithmetic operators to perform various operations. For example, users can input numbers, and the program can add, subtract, multiply, or divide them based on the user’s choice.
Example:
let num1 = 10;
let num2 = 2;
let operation = "+";
let result;
if (operation === "+") {
result = num1 + num2;
} else if (operation === "-") {
result = num1 - num2;
} else if (operation === "*") {
result = num1 * num2;
} else if (operation === "/") {
result = num1 / num2;
}
console.log(result); // Result: 12 (if operation is '+')
In this example, the program checks the value of operation
and
performs the corresponding arithmetic operation on num1
and
num2
.
Arithmetic operators are a vital part of JavaScript programming. They enable you to perform a wide range of mathematical operations, from basic addition to complex exponentiation. By understanding these operators and knowing when and how to use them, you can effectively manage numerical data and create powerful applications.
The + Operator for String and Number
The +
operator in JavaScript serves a dual purpose: it acts as an
arithmetic addition operator for numbers and a concatenation operator for
strings. This versatility makes it a key tool when working with different data
types. Understanding how the +
operator behaves is crucial for
avoiding unexpected results, especially when combining numbers and strings in
calculations or output formatting.
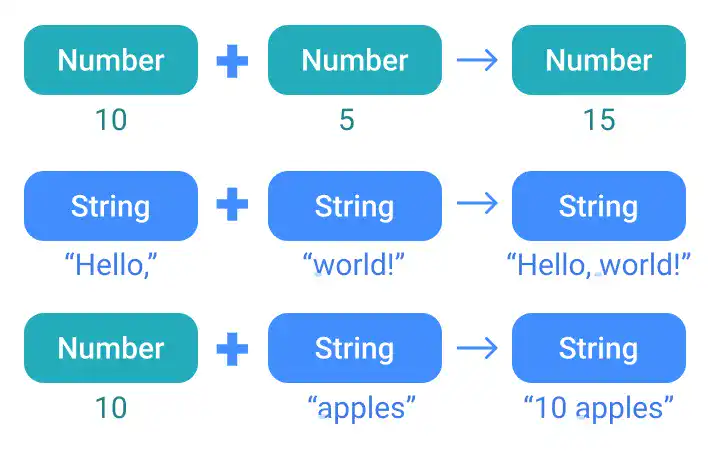
Using the "+" Operator for Numbers
When the +
operator is used with numbers, it behaves as expected:
it adds the two numbers together.
Example:
let x = 10;
let y = 5;
let result = x + y; // result is 15
Using the "+" Operator for Strings
However, when the +
operator is used with strings, it
concatenates them, meaning it joins the two strings together rather than
performing an arithmetic operation.
Example:
let str1 = "Hello, ";
let str2 = "world!";
let greeting = str1 + str2; // greeting is "Hello, world!"
Using the "+" Operator for Numbers and Strings
When the +
operator is used with a mix of numbers and strings,
JavaScript treats the number as a string and performs concatenation. This type
coercion can lead to unexpected results if you're not careful.
Example:
let num = 10;
let str = " apples";
let result = num + str; // result is "10 apples"
Reference Links:
FAQ: Arithmetic Operators in JavaScript
What Are Arithmetic Operators?
Arithmetic operators are fundamental tools in JavaScript that enable numerical calculations like addition (+), subtraction (-), multiplication (*), division (/), modulus (%), and exponentiation (**). These symbols work with integers and floating-point numbers to manipulate numeric data across various applications. They are essential for building logic and handling mathematical operations in JavaScript programs.
How is the Addition Operator (+) used in JavaScript?
The addition operator (+) is used to add two numbers. It can also be used to concatenate strings, making it versatile in both numerical and string operations. For example, adding 5 and 3 results in 8, while concatenating "Hello" and "World" results in "HelloWorld".
What are some use cases of Arithmetic Operators with Variables?
Arithmetic operators are often used with variables to store dynamic results. They can be used in various scenarios, such as performing operations on variables, calculating the area of a circle using the formula Area = π * r^2, or creating a simple calculator program that performs operations based on user input.
How does the + Operator work with Strings and Numbers?
The + operator in JavaScript serves a dual purpose: it acts as an arithmetic addition operator for numbers and a concatenation operator for strings. When used with numbers, it adds them together. When used with strings, it concatenates them. If used with a mix of numbers and strings, JavaScript treats the number as a string and performs concatenation, which can lead to unexpected results.
What is the Modulus Operator (%) used for?
The modulus operator (%) returns the remainder of a division operation. It’s useful for tasks like determining whether a number is even or odd. For example, 10 divided by 3 leaves a remainder of 1.
What should be considered when using the Division Operator (/) in JavaScript?
The division operator (/) divides one number by another. It's important to be cautious when dividing by zero, as it will result in Infinity or NaN (Not a Number). Proper error handling should be implemented to manage such cases.